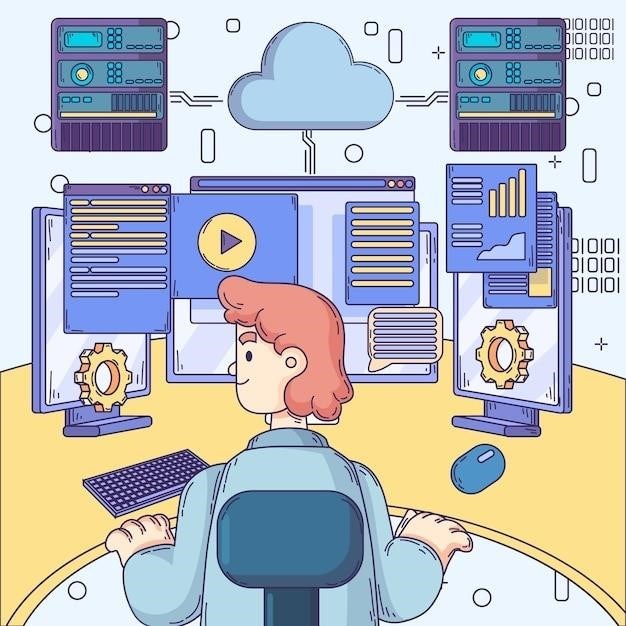
Automate the Boring Stuff with Python⁚ A Comprehensive Guide
This comprehensive guide delves into the world of Python automation, empowering you to streamline repetitive tasks and reclaim your precious time. Discover how Python, a versatile and beginner-friendly language, can automate everyday tasks, ranging from simple file management to complex web scraping and data manipulation. Join us on a journey to unlock the power of Python and automate the mundane, freeing you to focus on what truly matters.
Introduction⁚ The Power of Python for Automation
In today’s fast-paced world, time is a precious commodity. Many of us find ourselves bogged down by repetitive, mundane tasks that consume valuable hours. Imagine spending hours renaming files, updating spreadsheets, or scraping data from websites – tasks that drain our energy and leave us yearning for more fulfilling activities. Fortunately, Python, a versatile programming language known for its simplicity and readability, offers a powerful solution⁚ automation. With Python, you can transform tedious, time-consuming tasks into automated processes that run smoothly and efficiently, freeing you to focus on more creative and strategic endeavors. Python’s ability to automate tasks extends beyond simple file management. You can harness its power to automate web scraping, email and text message sending, PDF manipulation, and more. By learning Python, you unlock a world of possibilities, empowering yourself to take control of your workflow and reclaim your time.
Why Python?
Python stands out as the ideal language for automation due to its exceptional combination of features⁚
- Beginner-Friendly Syntax⁚ Python’s clear and concise syntax makes it remarkably easy to learn, even for those with no prior programming experience. Its readability resembles natural language, making it a joy to write and understand.
- Extensive Libraries⁚ Python boasts a vast collection of pre-built libraries, each tailored for specific tasks. These libraries offer ready-made tools for tasks like web scraping, data analysis, file manipulation, and more, significantly reducing development time and effort.
- Active Community⁚ Python has a thriving and supportive community of developers, ensuring ample resources, tutorials, and forums to aid your learning journey. This collaborative environment fosters continuous improvement and knowledge sharing.
- Cross-Platform Compatibility⁚ Python runs seamlessly on various operating systems, including Windows, macOS, and Linux, making it a versatile choice for diverse environments.
- Free and Open-Source⁚ Python is completely free to use and distribute, making it accessible to everyone. Its open-source nature allows for community contributions, ensuring its ongoing development and improvement.
These attributes make Python the perfect language for automating the boring stuff, enabling you to focus on higher-level tasks and achieve greater productivity.
Getting Started with Python
Embarking on your Python automation journey is surprisingly straightforward. Here’s a step-by-step guide to get you up and running⁚
- Download and Install Python⁚ Begin by downloading the latest version of Python from the official website (https://www.python.org/). The installation process is simple and intuitive, guiding you through the necessary steps.
- Choose an IDE or Text Editor⁚ An Integrated Development Environment (IDE) or a text editor provides a structured environment for writing and executing Python code. Popular choices include PyCharm, VS Code, and Sublime Text.
- Run Your First Program⁚ Open your chosen IDE or text editor and create a new file named “hello.py.” Inside the file, type the following code⁚
print("Hello, world!")
- Execute the Code⁚ Save the file and run it using your IDE or by opening a terminal and typing “python hello.py.” This will display “Hello, world!” in your terminal, marking your first successful Python program.
- Explore Online Resources⁚ Numerous online resources are available to support your learning. The official Python documentation (https://docs.python.org/3/), tutorials on websites like W3Schools (https://www.w3schools.com/python/), and interactive platforms like Codecademy offer excellent learning materials.
With these steps, you’ll be equipped to start writing your own Python automation programs.
Essential Python Concepts for Automation
To unlock the full potential of Python for automation, understanding a few key concepts is crucial. These concepts form the foundation of your automation journey, enabling you to write efficient and effective programs⁚
- Variables⁚ Think of variables as containers that hold data. They allow you to store and manipulate information within your programs; For instance, you might create a variable called “filename” to store the name of a file you want to process.
- Data Types⁚ Python supports various data types, each representing a different kind of information. Common data types include integers (numbers like 1, 2, 3), floats (decimal numbers like 3.14), strings (text like “hello”), and lists (ordered collections of items).
- Operators⁚ Operators perform actions on data. Arithmetic operators (like +, -, *, /) are used for calculations, while comparison operators (like ==, !=, >, <) are used for checking conditions.
- Conditional Statements⁚ Conditional statements, such as “if,” “else,” and “elif,” allow your program to make decisions based on specific conditions. This is essential for creating programs that can handle different scenarios.
- Loops⁚ Loops allow you to repeat a block of code multiple times. “For” loops iterate over a sequence of items, while “while” loops continue executing as long as a certain condition is true.
- Functions⁚ Functions are reusable blocks of code that perform specific tasks. Defining functions helps you organize your programs, make them more readable, and avoid code duplication.
Mastering these essential concepts will equip you with the building blocks to create powerful Python automation scripts.
Automating Repetitive Tasks
Let’s dive into the heart of Python automation⁚ tackling those tedious tasks that eat away at your time. Imagine a world where you can automate the mundane, freeing yourself to focus on more creative and engaging work; Python empowers you to do just that. Here are some common repetitive tasks that Python can handle effortlessly⁚
- File Renaming⁚ Say goodbye to manually renaming dozens of files. Python can easily rename files based on patterns, add prefixes or suffixes, and even change file extensions.
- Data Extraction⁚ Need to extract specific information from text files, spreadsheets, or even PDFs? Python’s powerful string manipulation tools and libraries like “re” (for regular expressions) can help you extract the data you need with ease.
- Web Scraping⁚ Python allows you to “scrape” data from websites, extracting valuable information like product prices, news articles, or social media posts. Libraries like “Beautiful Soup” and “requests” simplify the process.
- Email Automation⁚ Sending out automated emails is a breeze with Python. You can easily craft and send personalized emails, schedule emails to be sent at specific times, and even manage email attachments.
- Spreadsheet Manipulation⁚ Python libraries like “openpyxl” and “pandas” let you work with Excel spreadsheets with ease. You can automate tasks like creating new spreadsheets, adding data, formatting cells, and generating reports.
As you explore these examples, you’ll realize the vast potential of Python for automating tasks that would otherwise consume hours of your time. Let Python be your digital assistant, freeing you to focus on the things that truly matter.
Working with Files and Directories
Python provides a robust set of tools for managing files and directories, making it a powerful ally for automating tasks related to file organization, manipulation, and analysis. Let’s explore some key aspects of working with files and directories in Python⁚
- File Operations⁚ Python’s built-in functions like “open” allow you to read, write, and modify files with ease. You can open files in various modes (read, write, append), read their contents line by line, write new data to files, and even append data to existing files.
- Directory Navigation⁚ Python’s “os” module provides a comprehensive set of functions for navigating directories, creating new directories, deleting existing ones, and listing the contents of directories. You can use these functions to organize your files efficiently and automate file-related tasks.
- File Renaming and Copying⁚ Python simplifies file renaming and copying. The “os.rename” function allows you to rename files, while “shutil.copy” enables you to create copies of files or entire directories. These functions empower you to manage your files with precision and speed.
- File Searching and Filtering⁚ Python’s “glob” module provides powerful pattern-matching capabilities for searching files and directories. You can use wildcards to search for files based on their names, extensions, or other criteria.
- File Handling Errors⁚ It’s crucial to handle potential errors when working with files. Python’s exception handling mechanisms allow you to gracefully handle situations like file-not-found errors, permission issues, or read/write errors, ensuring your scripts run smoothly.
By mastering these file and directory manipulation techniques, you can create Python scripts that streamline your file management workflows, saving you time and effort. From organizing your documents to automating backups, Python can be your ultimate file management companion.
Web Scraping with Python
Web scraping, the process of extracting data from websites, is a powerful technique for gathering information, analyzing trends, and automating tasks. Python, with its rich set of libraries, makes web scraping a relatively straightforward endeavor. Let’s explore the key elements of web scraping with Python⁚
- Libraries for Web Scraping⁚ Python offers several specialized libraries for web scraping, including⁚
- Requests⁚ This library simplifies the process of sending HTTP requests to websites, fetching web pages, and handling responses.
- Scrapy⁚ For more complex web scraping tasks, Scrapy provides a framework for building robust, efficient web scrapers.
By combining these libraries and techniques, you can build Python scripts to automate web scraping tasks, collecting valuable data from websites for analysis, research, or personal projects. Web scraping opens a world of possibilities for data extraction and automation.
Automating Email and Text Messages
Python empowers you to automate email and text message sending, simplifying communication tasks and streamlining workflows. Imagine sending automated reminders, notifications, or even personalized messages with just a few lines of code. Let’s explore how Python can enhance your communication capabilities⁚
- Email Automation⁚
- smtplib⁚ Python’s built-in smtplib library allows you to send emails using the Simple Mail Transfer Protocol (SMTP). You can configure the library with your email provider’s settings (e.g., Gmail, Outlook) to send emails programmatically.
- Email Templates⁚ For more sophisticated email formatting, you can utilize libraries like Jinja2 or String.Template to create reusable email templates. These templates can be dynamically populated with data, allowing you to send personalized emails.
- Attachments⁚ Python allows you to attach files to emails, making it easy to send documents, reports, or images with your messages.
- Text Message Automation⁚
- Twilio⁚ Twilio is a popular cloud communication platform that provides APIs for sending SMS messages. You can integrate Twilio’s API with your Python script to send text messages.
- Other Providers⁚ Similar to Twilio, other cloud messaging providers (e.g., Nexmo, SendGrid) offer APIs for sending SMS messages.
- Text Message Templates⁚ You can use text message templates to create reusable message structures and personalize them with dynamic data.
By leveraging these libraries and services, you can automate email and text message sending, saving time and effort in your communication tasks. Python provides the tools to streamline your communication workflows and enhance your productivity.
Working with Spreadsheets and Data
Python excels at working with spreadsheets and data, offering a powerful set of tools to automate tasks, analyze information, and create insightful reports. Whether you’re dealing with simple spreadsheets or large datasets, Python provides the flexibility and efficiency to handle your data manipulation needs. Let’s explore how Python can transform your spreadsheet and data workflows⁚
- Openpyxl⁚ This library allows you to read, write, and manipulate Excel spreadsheets (.xlsx files). You can create new spreadsheets, access existing data, modify cells, format content, and even insert charts.
- Pandas⁚ A cornerstone of data analysis with Python, Pandas provides a powerful DataFrame structure for working with tabular data. DataFrames are like enhanced spreadsheets, allowing you to perform⁚
- Data Cleaning⁚ Remove duplicates, handle missing values, and transform data to a consistent format.
- Data Analysis⁚ Perform calculations, aggregations, and statistical analysis on your data.
- Data Visualization⁚ Combine Pandas with libraries like Matplotlib or Seaborn to generate informative charts and graphs.
- CSV⁚ Python’s built-in csv module enables you to work with Comma-Separated Value (CSV) files. You can read data from CSV files, write data to CSV files, and even manipulate the data within these files.
- Other Formats⁚ Python supports working with various data formats, including JSON, XML, and more, allowing you to import, process, and export data in a wide range of formats.
By utilizing these libraries, you can automate repetitive tasks, analyze data, and generate insightful reports efficiently. Python empowers you to take control of your spreadsheet and data workflows, making data-driven decisions with ease.
Automating PDF Manipulation
PDF files are ubiquitous, and often require manual manipulation. Python offers a solution for automating these tasks, saving you time and effort; Let’s explore the capabilities of Python for working with PDFs⁚
- PyPDF2⁚ This library provides a robust set of tools for extracting text, images, and metadata from PDF files. You can also merge, split, and rotate PDFs.
- PDFMiner⁚ Another powerful library for PDF manipulation. PDFMiner excels at extracting text content from PDFs, even when the text is embedded within images or complex layouts.
- ReportLab⁚ While not strictly for PDF manipulation, ReportLab is a library for creating PDF documents from scratch. You can use it to generate reports, invoices, and other documents with customized layouts and content.
- Other Libraries⁚ Several other libraries exist for PDF manipulation, each with its own strengths and weaknesses.
With Python’s PDF libraries, you can⁚
- Extract Text⁚ Retrieve text content from PDFs for analysis or data extraction.
- Extract Images⁚ Extract images from PDFs to use in other applications.
- Merge PDFs⁚ Combine multiple PDFs into a single document.
- Split PDFs⁚ Divide a single PDF into multiple smaller PDFs.
- Rotate PDFs⁚ Change the orientation of pages within a PDF.
- Add Watermarks⁚ Apply watermarks to PDFs for security or branding purposes.
- Encrypt PDFs⁚ Protect PDFs with passwords to restrict access.
Python’s PDF manipulation capabilities make it a valuable tool for streamlining workflows that involve interacting with PDF files. Whether you’re dealing with large volumes of documents or need to automate repetitive tasks, Python provides the power and flexibility to handle your PDF needs.